C++ 在 C 语言的基础上增加了面向对象编程,C++ 支持面向对象程序设计。类是 C++ 的核心特性,通常被称为用户定义的类型。
类用于指定对象的形式,它包含了数据表示法和用于处理数据的方法。类中的数据和方法称为类的成员。函数在一个类中被称为类的成员。
类定义
类定义是以关键字 class
开头,后跟类的名称。类的主体是包含在一对花括号中。类定义后必须跟着一个分号或一个声明列表。
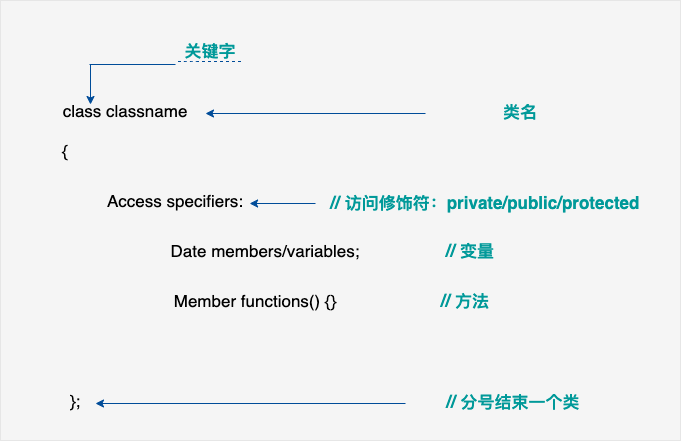
实例:
1 2 3 4 5 6 7
| class Box { public: double length; double breadth; double height; };
|
关键字 public
,private
或 protected
确定了类成员的访问属性。
定义对象
声明类的对象,与声明基本类型的变量一样。
访问数据成员
类的对象的公共数据成员可以使用直接成员访问运算符 .
来访问。
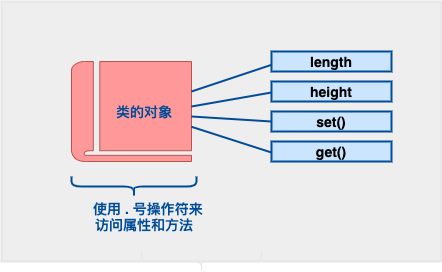
实例
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57
| #include <iostream>
using namespace std;
class Box { public: double length; double breadth; double height; double get(void); void set(double len, double bre, double hei); };
double Box::get(void) { return length * breadth * height; }
void Box::set(double len, double bre, double hei) { length = len; breadth = bre; height = hei; } int main() { Box Box1; Box Box2; Box Box3; double volume = 0.0;
Box1.height = 5.0; Box1.length = 6.0; Box1.breadth = 7.0;
Box2.height = 10.0; Box2.length = 12.0; Box2.breadth = 13.0;
volume = Box1.height * Box1.length * Box1.breadth; cout << "Box1 的体积:" << volume << endl;
volume = Box2.height * Box2.length * Box2.breadth; cout << "Box2 的体积:" << volume << endl;
Box3.set(16.0, 8.0, 12.0); volume = Box3.get(); cout << "Box3 的体积:" << volume << endl; return 0; }
|
结果:
1 2 3
| Box1 的体积:210 Box2 的体积:1560 Box3 的体积:1536
|
注意:私有的成员和受保护的成员不能使用直接成员访问运算符 .
来直接访问。
C++ 类&对象